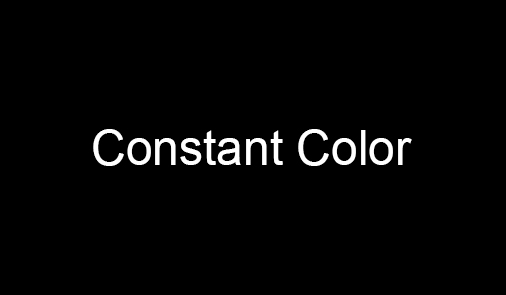
Constant Color
A simple pure shaded color.
Learn moreA collection of shader and experiments using OpenGL Shading Language. Here you are going to find:
If you want to know more, please consider vising the repository.
Constant shaded color and not affected by lighting. It is probably the most basic shader to produce.
A simple pure shaded color.
Learn moreA simple pure shaded color from texture.
Learn moreA simple iridescence effect inspired by the Goniochromism phenomenon, which varies its aspect based on the relationship between the surface normal and the camera angle. The visual beautification is due the pseudo noise function, that makes the aspect a little more appealing.
float NaiveNoise(vec3 freq, vec3 offset) {
// Naive noise function to make irregularity
return sin(2.0*PI*P.x*freq.x*2.0 + 12.0 + offset.x) +
cos(2.0*PI*P.z*freq.x + 21.0 + offset.x) *
sin(2.0*PI*P.y*freq.y*2.0 + 23.0 + offset.y) +
cos(2.0*PI*P.y*freq.y + 32.0 + offset.y) *
sin(2.0*PI*P.z*freq.z*2.0 + 34.0 + offset.z) +
cos(2.0*PI*P.x*freq.z + 43.0 + offset.z);
}
vec3 iridescence(
float orient,
float noiseMult,
vec3 freqA,
vec3 offsetA,
vec3 freqB,
vec3 offsetB
) {
// This function returns a iridescence value based on orientation
vec3 irid;
irid.x = abs(cos(
2.0*PI*orient*freqA.x + NaiveNoise(freqB, offsetB)*noiseMult + 1.0 + offsetA.x
));
irid.y = abs(cos(
2.0*PI*orient*freqA.y + NaiveNoise(freqB, offsetB)*noiseMult + 2.0 + offsetA.y
));
irid.z = abs(cos(
2.0*PI*orient*freqA.z + NaiveNoise(freqB, offsetB)*noiseMult + 3.0 + offsetA.z
));
return irid;
}
A simple iridescent shader.
Learn more